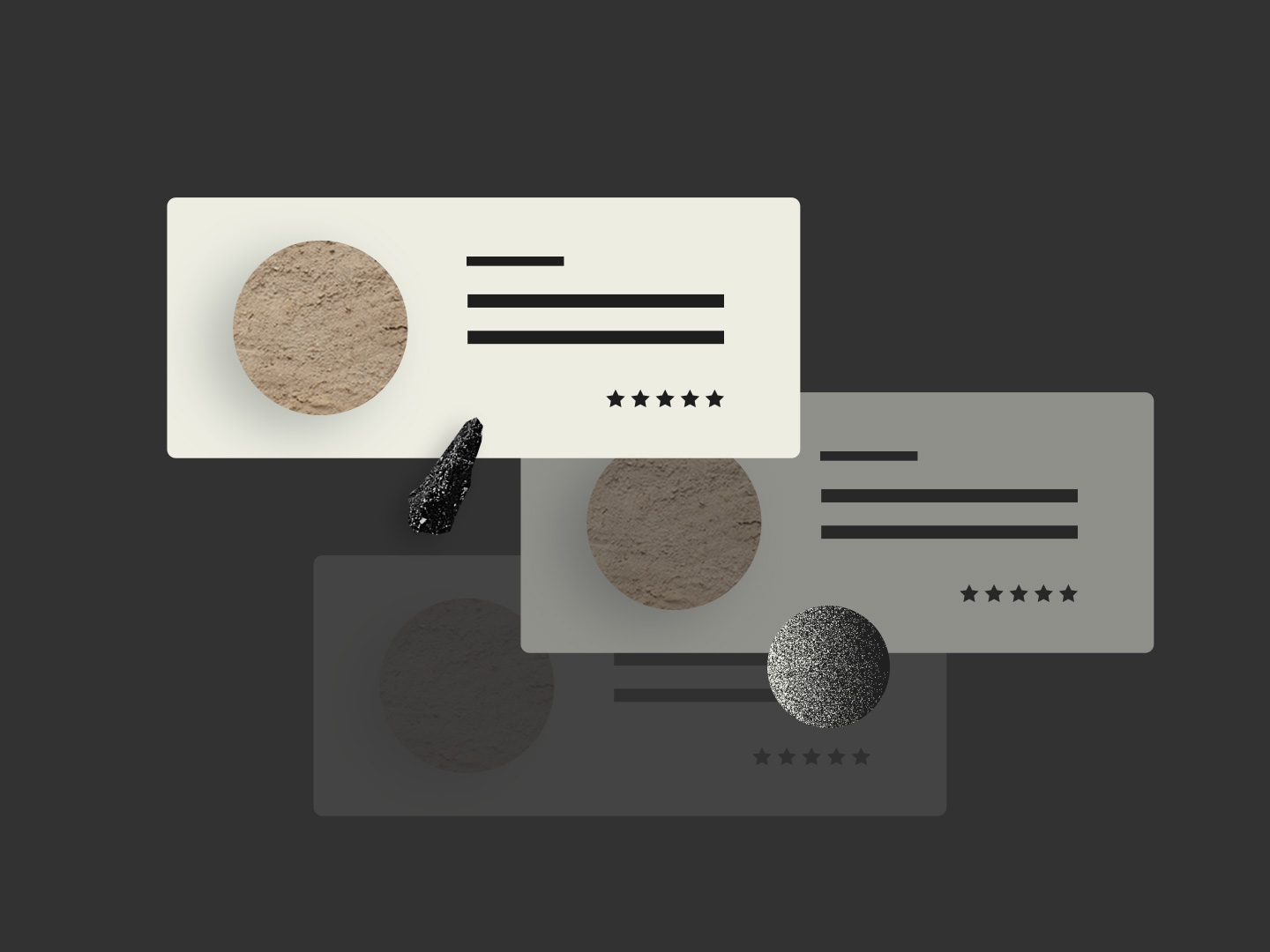
In this tutorial, I’ll guide you step by step to integrate and display Google My Business (GMB) information on your website, such as address, opening hours, phone number, ratings, and reviews.
We will use the Google Places API and JavaScript to retrieve and dynamically show this information.
NOTE: Google My Business has a limit of 5 reviews, i.e. only latest 5 reviews will be displayed on your site.
Prerequisites:
- Google Cloud Account: You’ll need a Google API key that allows access to the Places API.
- Access to Google My Business: You should have access to the GMB listing of the business you want to retrieve information from.
Step 1: Including the Google Places API
First, we need to include the Google Places library in your project. Add the following script to the <head>
of your HTML file:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places"></script>
Make sure to replace YOUR_API_KEY
with your valid API key.
Step 2: HTML Structure
In the body of your HTML, you’ll need a container where the Google My Business information will be displayed. Create a div
with a specific ID for this purpose, for example:
<div id="map"></div>
<div id="google-reviews">
<!-- Google My Business information will be displayed here -->
<div id="loading">Loading...</div>
</div>
This div
will be where the GMB content, such as reviews, address, and ratings, is dynamically added.
Step 3: Creating the Script to Fetch Information
Now we will write the JavaScript code that interacts with the Google Places API. The following code will request and display the information from your GMB listing.
let request = {
placeId: 'ChIJjXbg66azUQ0RyJ8NMTZCq5A', // Replace with your business's Place ID
};
service = new google.maps.places.PlacesService(map);
service.getDetails(request, pom_places_callback);
function pom_places_callback(place, status) {
if (status == google.maps.places.PlacesServiceStatus.OK) {
const googleReviewsDiv = document.getElementById('google-reviews');
googleReviewsDiv.innerHTML = ''; // Clear the loading message
// Basic place information
const isOpen = place.opening_hours && place.opening_hours.isOpen();
const placeInfoDiv = document.createElement('div');
placeInfoDiv.innerHTML = `
<p><strong>Address:</strong> ${place.adr_address}</p>
<p><strong>Open Now:</strong> ${isOpen ? "Yes" : "No"}</p>
<p><strong>Opening Hours:</strong></p>
<ul>
${place.opening_hours.weekday_text.map(day => `<li>${day}</li>`).join('')}
</ul>
<p><strong>Phone Number:</strong> ${place.formatted_phone_number}</p>
<p><strong>Rating:</strong> ${place.rating} / 5 (based on ${place.user_ratings_total} reviews)</p>
<p><a href="${place.url}" target="_blank">See on Google Maps</a></p>
`;
// Reviews
const reviewsDiv = document.createElement('div');
reviewsDiv.classList.add('row');
place.reviews.forEach(review => {
const reviewDiv = document.createElement('div');
reviewDiv.classList.add('col-md-4');
reviewDiv.style.marginBottom = '20px';
const starRating = createStarRating(review.rating);
reviewDiv.innerHTML = `
<img src="${review.profile_photo_url}" alt="${review.author_name}" style="border-radius: 50%; width: 50px; height: 50px; margin-right: 10px;">
<div>
<p><strong>${review.author_name}</strong><br><small>${review.relative_time_description}</small><br>${starRating}</p>
<p>${review.text}</p>
</div>
`;
reviewsDiv.appendChild(reviewDiv);
});
// Add more reviews info
const afterReviewDiv = document.createElement('div');
afterReviewDiv.classList.add('col-md-4');
afterReviewDiv.style.marginBottom = '20px';
afterReviewDiv.innerHTML = `
<div>
<p><strong>More on Google</strong></p>
</div>
`;
reviewsDiv.appendChild(afterReviewDiv);
googleReviewsDiv.appendChild(placeInfoDiv);
googleReviewsDiv.appendChild(reviewsDiv);
}
}
This script does several key things:
- Request business information: Using the
placeId
, the API retrieves detailed information about the business. - Display dynamic information: Data such as the address, if the business is currently open, opening hours, phone number, ratings, and reviews are displayed dynamically.
- Star ratings: Reviews include stars representing the business’s rating, with a system to show both full and half stars.
Step 4: Function to Display Star Ratings
The createStarRating
function handles the visual representation of the business’s rating in the form of stars. Add this code to your JavaScript file:
function createStarRating(rating) {
const fullStars = Math.floor(rating);
const halfStar = rating % 1 >= 0.5 ? 1 : 0;
const emptyStars = 5 - fullStars - halfStar;
let starsHtml = '';
for (let i = 0; i < fullStars; i++) {
starsHtml += '<span>★</span>';
}
if (halfStar) {
starsHtml += '<span>★☆</span>';
}
for (let i = 0; i < emptyStars; i++) {
starsHtml += '<span>☆</span>';
}
return starsHtml;
}
This function calculates how many full stars, half stars, and empty stars should be displayed.
Step 5: Customization
- Changing the
placeId
: TheplaceId
is the unique identifier for your business on Google My Business. You need to replace'ChIJjXbg66azUQ0RyJ8NMTZCq5A'
with the Place ID of your business. You can find your Place ID here. - Styling: You can customize the style of the reviews, change the HTML structure, or add your own CSS styles to better fit your design.
Step 6: Execution
Save your HTML file and open the page in your browser. You’ll see the information from your business, including Google reviews, dynamically displayed in the reviews div
we created earlier.
This is how it will look like:
Conclusion
This tutorial has shown you how to integrate and display Google My Business information on your website using the Google Places API.
You can now customize it based on your business needs and adapt it to improve the user experience on your site.
If you have additional questions, check out the official Google Places API documentation.